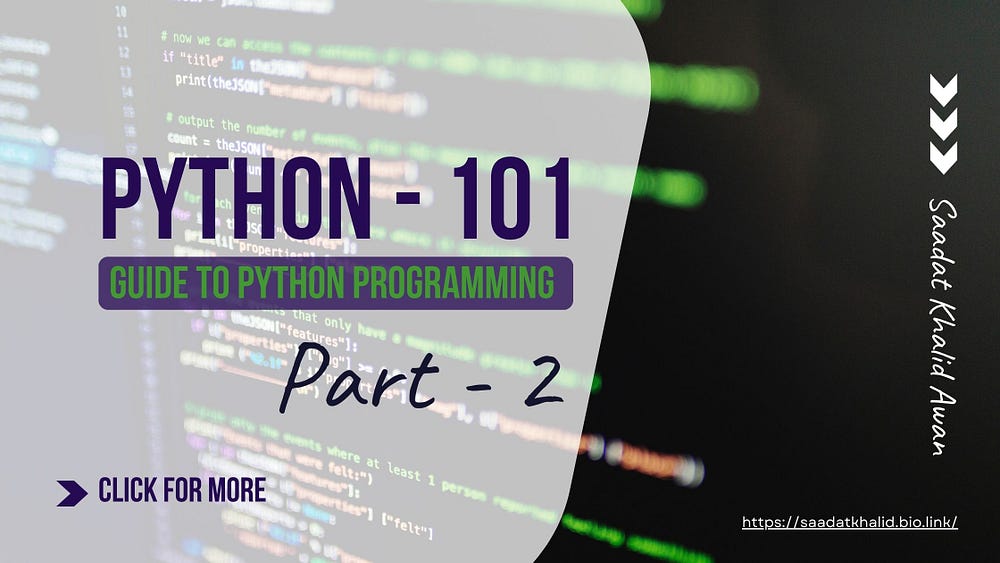
Welcome to the next blog in our Python 101 Blog Series! You can check this blog [Python 101 — Guide to Python Programming] if you missed the first part.
Let’s dive into the topic:
1. Operators in Python
Today, we’ll explore Operators, which allow us to perform mathematical operations like addition, subtraction, multiplication, and more.
1. Addition (+)
Addition is used to add the numbers.
print(5 + 5)
# Output: 10
2. Subtraction (-)
Subtraction is used to calculate the difference between numbers.
print(5 - 2)
# Output: 3
3. Multiplication (*)
Multiplication gives the product of two numbers.
print(5 * 5)
# Output: 25
4. Division (/)
Division divides one number by another.
print(10 / 2)
# Output: 5.0
5. Modulus (%)
The modulus operator gives the remainder when one number is divided by another.
print(10 % 3)
# Output: 1
6. Floor Division (//)
Floor division gives the quotient, excluding the decimal part.
print(10 // 3)
# Output: 3
7. Power ()**
The power operator raises a number to the power of another.
print(3 ** 2)
# Output: 9
Complex Expressions
In Python, expressions are evaluated using the PEMDAS rule:
- Parentheses
- Exponents
- Multiplication
- Division
- Addition
- Subtraction
It evaluates from left to right for operators of the same precedence.
print(3**2 / 2 * 3 / 3 + 6 - 4)
# Output: 7.5
2. Strings
Strings are text enclosed in single, double, or triple quotes.
print('Single Quotes')
print("Double Quotes")
print('''Triple Quotes''')
Task: How to write this: Hello, What’s up?
3. Variables
Variables are containers that hold data. Python automatically determines the type of a variable based on its value.
number = 10
# Here, the value 10 is assigned to the variable `number`.
Types of Variables
- Numeric: Numbers like 10 or 3.14
- String: Text like “Hello”
- Boolean: True or False
Bonus: Check a variable’s type using the type()
function:
print(type(number))
# Output: <class 'int'>
Rules for Naming Variables
- Use letters, numbers, or underscores.
- Don’t start with a number.
- Avoid reserved keywords like
if
,else
, etc. - Don’t use spaces in variable names.
4. Input Variables
Input variables allow us to take user input and store it in a variable.
name = input("What is your name? ")
greeting = "Hello"
print(greeting, name)
# If the user enters "Saadat", the output will be: Hello Saadat
Note: The input()
function always returns a string. Convert it to another type if needed.
5. Conditional Operators
Conditional operators are used to compare values.
print(10 == 10) # Equal to
print(10 != 5) # Not equal to
print(10 < 15) # Less than
print(10 > 5) # Greater than
print(10 >= 10) # Greater than or equal to
print(10 <= 20) # Less than or equal to
Simple Program:
age_of_student = 4
required_age_at_school = 5
print(age_of_student >= required_age_at_school)
# Output: False
6. Type Conversion
Type conversion is used to convert a variable from one type to another.
Implicit Conversion
Python does this automatically where possible.
x = 10 + 2.5
print(type(x))
# Output: <class 'float'>
Explicit Conversion
You can convert types manually using int()
, float()
, or str()
.
age = input("Enter your age: ")
age = int(age) # Converts the string input into an integer
7. If, Else, and Elif Statements
These are used to check conditions in your program. If the condition is `True` the block of code under the if will execute, if the condition is `False` then the else part will be executed. The elif is used where you need to add extra conditions.
if student_score >= 60:
print("Congrats! You passed.")
elif student_score == 50:
print("You barely passed. Work harder!")
else:
print("You failed. Better luck next time!")
8. Loops
Loops are used to repeat a block of code. The loop will execute until the condition/limit is hit.
There are two types of loops `while` and `for`.
while loop:
x = 1
while x <= 10:
print(x)
x += 1
for loop:
for x in range(1, 11):
print(x)
Breaking a loop:
fruits = ['Apple', 'Banana', 'Orange', 'Mango']
for fruit in fruits:
print(fruit)
if fruit == 'Orange':
break
9. Functions
Functions are blocks of code designed to perform specific tasks.
Built-in Functions:
Examples: print()
, type()
, len()
User-Defined Functions:
def greet(name):
print("Hello", name)
greet("Saadat")
# Output: Hello Saadat
10. Importing Libraries
Libraries extend Python’s capabilities. Examples: math
, statistics
, sklearn
.
import math
import statistics
print("Value of Pi:", math.pi)
numbers = [10, 20, 30, 40]
print("Average:", statistics.mean(numbers))