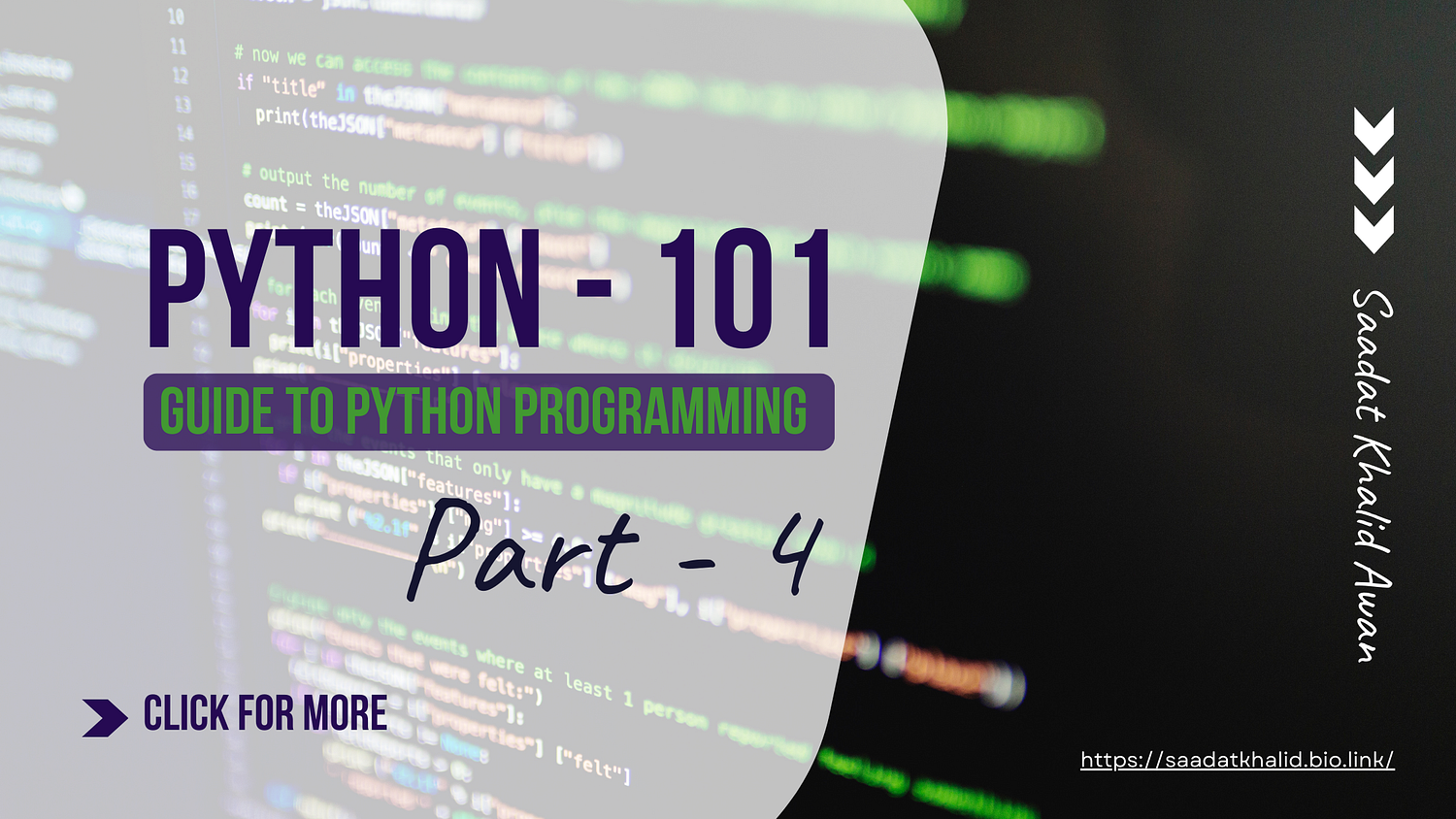
Welcome to Part 4 of the Python 101 Series!
If you’ve missed the previous parts, don’t worry. You can check out Part 1, Part 2, and Part 3 to catch up.
In this blog, we’ll cover the following topics:
- Lambda Functions
- Exception Handling
- File Handling
Let’s dive right in!
1. Lambda Functions
A lambda function is a small, anonymous function in Python. It can take multiple arguments but is restricted to a single expression. Lambda functions are often used for short-term tasks and are written in a single line.
lambda arguments: expression
# Adding 10 to a number
number = lambda num: num + 10
print(number(5)) # Output: 15
# Multiplying two numbers
x = lambda a, b: a * b
print(x(5, 6)) # Output: 30
Exception Handling
Exception handling ensures your program doesn’t crash when errors occur. Instead of abruptly stopping, Python allows you to handle errors gracefully and continue running the program.
Key Components of Exception Handling:
try
: Tests a block of code for errors.except
: Handles the error if one occurs.else
: Executes code if no error occurs.finally
: Executes code regardless of whether an error occurred or not.
Example: Basic Exception Handling
try:
print(x) # 'x' is not defined
except:
print("An exception occurred") # Output: An exception occurred
Example: Handling Specific Errors
try:
print(x)
except NameError:
print("Variable x is not defined") # Output if NameError occurs
except:
print("Something else went wrong") # Output for other errors
Example: Using else
The else
block runs only when no error occurs in the try
block.
try:
print("Hello")
except:
print("Something went wrong")
else:
print("Nothing went wrong") # Output: Nothing went wrong
Example: Using finally
The finally
block runs no matter what.
try:
print(x) # 'x' is not defined
except:
print("Something went wrong")
finally:
print("The 'try except' block is finished") # Always executes
File Handling
File handling allows you to create, read, write, append, and delete files in Python.
Opening Files with open()
The open()
function is used to work with files. It requires two parameters:
- Filename: The name of the file.
- Mode: Specifies the purpose (read, write, etc.).
File Modes:
r
: Read mode (Error if the file doesn’t exist).a
: Append mode (Creates the file if it doesn’t exist).w
: Write mode (Creates the file if it doesn’t exist).x
: Create mode (Error if the file already exists).
Example: Reading a File
file = open("textfile.txt", "r")
print(file.read())
file.close() # Always close the file after working with it
Example: Appending to a File
file = open("textfile.txt", "a")
file.write("Now the file has more content!")
file.close()
# Reading the updated file
file = open("textfile.txt", "r")
print(file.read())
Example: Deleting a File
To delete a file, you’ll need the os
module.
import os
# Deleting a file
os.remove("textfile.txt")
Key Notes:
- Always close files after working with them using
file.close()
. - File handling errors can be managed using exception handling for a smoother workflow.
That’s it for Part 4 of the Python 101 Series! Keep practicing these concepts to master them.
If you have any questions, drop them in the comments!